CWU Fitlab
CRUD web application using Streamlit
Overview
CWU Fitlab is a full-stack web application created for an upper level practicum for seniors at Central Washington University. CWU FitLab is a comprehensive fitness assessment experience provided by trained CWU students pursuing a degree in Exercise Science. This application makes it easier to manage client scheduling, track client progress over time, and provide access to client assessment data.
Tech Stack
- 🐍 Python 🐍
- HTML5
- CSS
- Google Cloud Platform
- Firebase
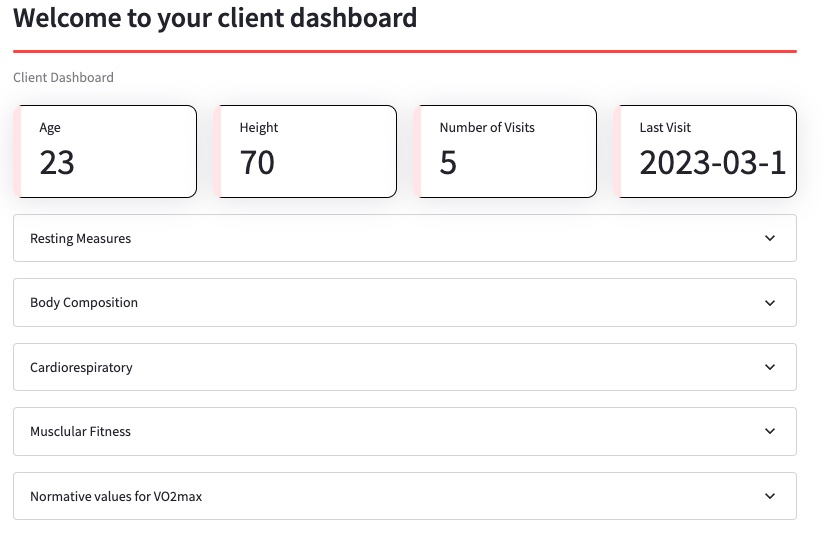
Data Science Packages
- 🐼 Pandas 🐼
- NumPy
- Matplotlib
- Seaborn
- Plotly
- Plotly Express
Notable Features
- Dashboard for clients to track progress over time
- Password protected login
- Update Firebase backend from “Technician” tab
- Calendly calendar to schedule visits
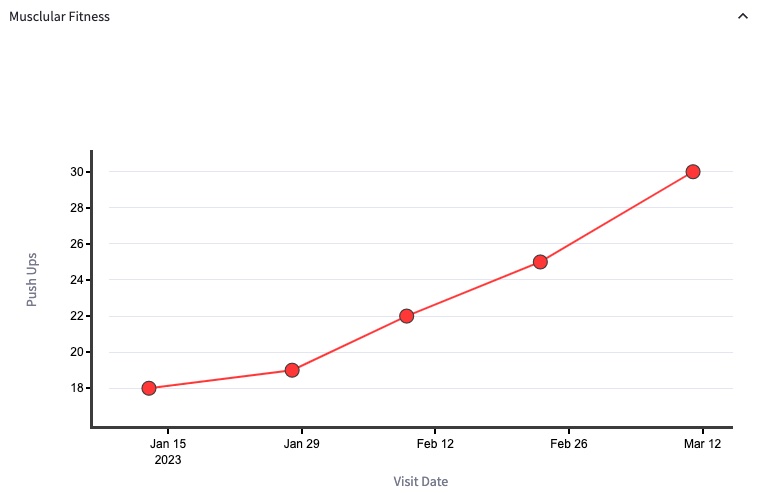
Code sample
Code sample of a function that fetches client data from the backend and creates a pandas dataframe to display on the technican page.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
def fetch_agg_data():
collection_ref = db.collection("clients")
subcollections = collection_ref.list_documents()
client_list = []
# Create pandas df to export as csv
df = pd.DataFrame()
# fetch client ID's and append to list
for subcollection in subcollections:
client_list.append(subcollection.id)
for c in client_list:
data = fetch_client_data(c)
data["Client"] = c
df = pd.concat([df, data], axis=0)
df.index = range(0,len(df))
return df